Camera Shake Effect In Unity
by Toys of Hendrik
Yesterday, when I was on meeting with Anoman Team talking about our new game and one of them giving an advice about the game feel of action games. The game feel are some additional effect like bloody particle effect, camera shake, a little shaking bullet, and etc. For example, you can play super crate box. Then he recommended this video to watch.
So basicly, this tutorail is very simple and short. You just use a built-in function calledRandom.InsideUnitSphere in the script and attach it to the camera. A little bit tricky because you must attach the camera as a child in empty object which have the same position with the camera. Then we can follow the steps.
1. Facing The Script
Ok, just make a script and name it CameraShake.cs and you can retype the script below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | using UnityEngine; using System.Collections; public class CameraShake : MonoBehaviour { public float shake = 0; public float shakeAmount = 0.7f; public float decreaseFactor = 1; // Use this for initialization void Start () { } // Update is called once per frame void Update () { if (shake > 0) { this.transform.localPosition = Random.insideUnitSphere * shakeAmount; shake -= Time.deltaTime * decreaseFactor; } else { shake = 0; } } } |
You see the “this.transform.localPosition” ? That shakes the camera locally around inside unit sphere in each frame of “shake” parameter decreases over time. The “shakeAmount” parameter is used for the shake range of inside unit sphere. “decreaseFactor” is like a x seconds and used to decreasing the “shake” parameter.
2. Attach It To The MainCamera Object
Drag and drop that script to the default main camera object.
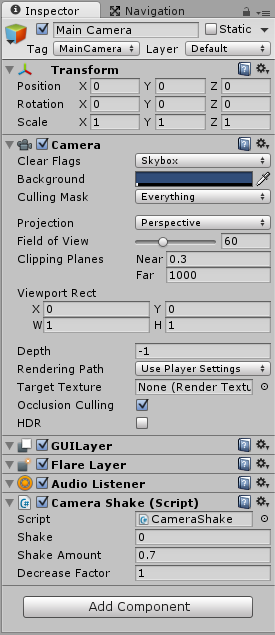
MainCamera Inspector
After that, try some play and see what happen.
3. Give The Trigger To Shake
Nothing happen when you clicked play last time because you haven’t give a trigger on “shake” parameter. Or if you want to shake it, simply make a trigger which can set “shake” to 1.0 or 2.0 or something like that and it starts to shake. For example we use when we press space bar, shake parameter value is 1 and the code is if(Input.GetKeyDown(KeyCode.Space)){shake=1;} add it inside Update method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | using UnityEngine; using System.Collections; public class CameraShake : MonoBehaviour { public float shake = 0; public float shakeAmount = 0.7f; public float decreaseFactor = 1; // Use this for initialization void Start () { } // Update is called once per frame void Update () { if(Input.GetKeyDown(KeyCode.Space)){ shake = 1; } if (shake > 0) { this.transform.localPosition = Random.insideUnitSphere * shakeAmount; shake -= Time.deltaTime * decreaseFactor; } else { shake = 0; } } } |
Click Play, press SpaceBar and you will see the camera position is move to the center of the world position or the real insideUnitSphere.
4. Tricking The insideUnitSphere Position
This is the last step and I believe you must be bored of this long post
LOL. So for the trick, we can create an empty game object (GameObject -> Create Empty) and set the position same as the Main Camera position. Inside that empty game object, it also have the Unit Sphere which can followed by MainCamera if we attach it as a child in empty game object.
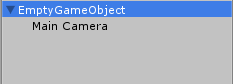
Move as a child.
Click Play and press SpaceBar, so that’s it. If you still have an scary error on your console you don’t need to worry about asking me 
