Explosion Force in Unity
by Toys of Hendrik
Explosion effect is one of the game feel part, it’ll be great if you make more cinematic. Post below is the example of simple explosion effect which I implemented on our new game, Orbiz.
So after you watch the short video above, we will know about how to make simple explosion force effect in unity. It means that you will watch flying objects which near explosion.
1. Open Unity
I know since I heard unreal 4 being free, so does unity 5 and another game engine too. But I still use unity 4.6 for a technical reason of my recent project. So if you already click it, make sure you can wait this splash screen.
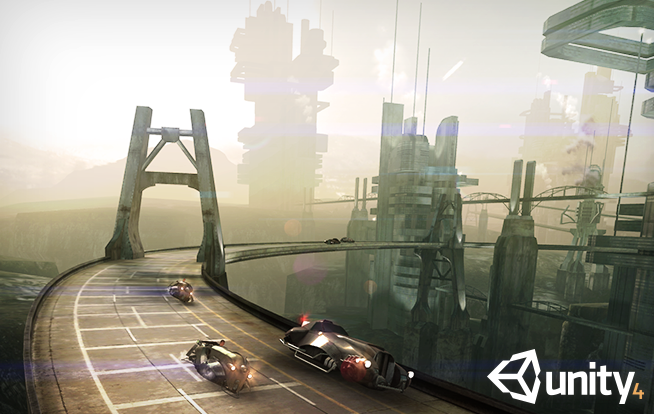
Superb Splash Screen
2. Set the Arena
Okay I know, just make a simple arena like this by cubes (GameObject –> 3D Object –> cube). Each cube should have a rigidbody and collider (Box Collider). It’s for cube which can explode, some cube for action flying and for the floor.
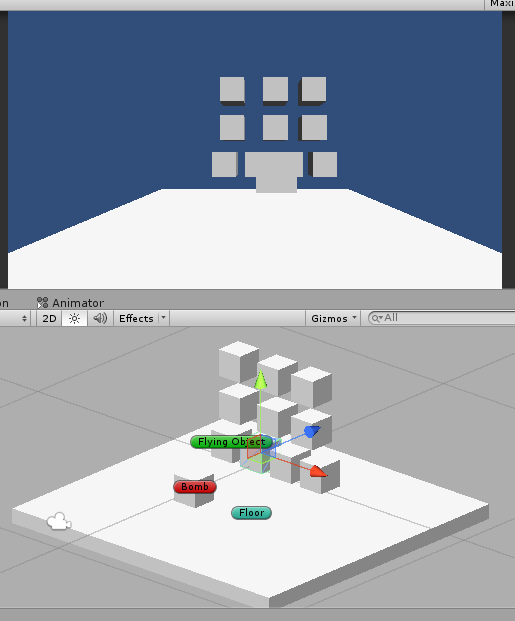
Before play.
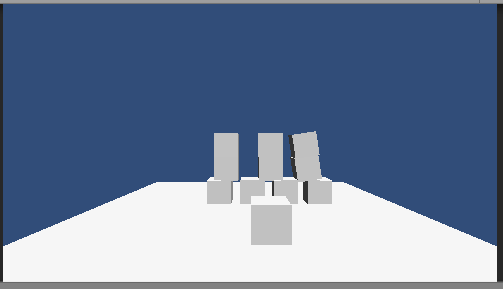
After play.
Give all of the cubes rigidbody except floor.
3. The Script
So after we set it up, let’s make a script which have an explode ability by a radius when it hit an “Cube” tag objects.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | using UnityEngine; using System.Collections; public class ExplodeExample : MonoBehaviour { public float radius = 5.0F; public float power = 10.0F; public float lift = 30; public float speed = 10; public bool explode = false; void FixedUpdate() { if(explode){ Vector3 explosionPos = transform.position; Collider[] colliders = Physics.OverlapSphere(explosionPos, radius); foreach (Collider hit in colliders) { if (hit.rigidbody) { hit.rigidbody.AddExplosionForce(power, explosionPos, radius, lift); } } } } void OnCollisionEnter(Collision collision) { if(collision.gameObject.tag == "Cube"){ explode = true; } } } |
We use lift, power, and radius for the AddExplosionForce parameter. Also a boolean variable if it’s hit an object, then the explode boolean sets true and send to Update() method. Attach the script into Bomb object and hit Play. It can’t explode automaticaly because the bomb object is far from the “Cube” tag objects, so we can move manually using Scene panel. But if we want that object moving forward by pressing keyboard key, just add these script on Update method.
if(Input.GetKey(KeyCode.UpArrow)){
transform.Translate(Vector3.forward * speed * Time.deltaTime);
}
transform.Translate(Vector3.forward * speed * Time.deltaTime);
}
4. Particle Effect
If you want explosion particle effect, import the built-in asset by following Assets –> Import Package –> Particles and if you watch the detail, click all and import.
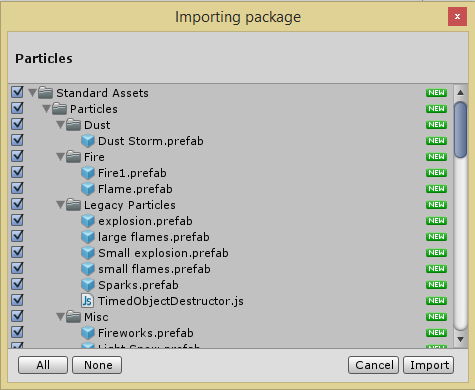
Import particles.
The sound ? you can download here via dropbox. So after the importing package done, you see the explosion prefab under Standart Assets –> particles –> Legacy Particles. Drag and drop to the hierarchy. You will see a javascript component calle TimedObjectDestructor. Should we convert into Csharp ? It’s okay, because we need an audio clip for the sound effect. So create new CSharp script called TimedObjectDestrucor.cs.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | using UnityEngine; using System.Collections; [RequireComponent(typeof(AudioSource))] public class TimedObjectDestructor : MonoBehaviour { public float timeOut = 1; public AudioClip soundEffect; // Use this for initialization void Awake() { Invoke("DestroyNow", timeOut); } void Start() { audio.PlayOneShot(soundEffect); } void DestroyNow() { Destroy(gameObject); } } |
We play explosion sound effect by adding audio.PlayOneShot(soundEffect) on Start() method. So we wait 1 seconds timeOut and will call DestroyNow() method. Attach that script into explosion prefab, but beffore attach delete the javascript component first. After attaching, drag and drpo explode sound that downloaded before into Sound Effect parameter at explosion Inspector, then move the explosion object from Hierarchy to Project panel so it will be a new prefab which we Instantiate by ExplodeExample script. About Instantiate, we should add Instantiate and Destroy Function inside ExplodeExample script.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | using UnityEngine; using System.Collections; public class ExplodeExample : MonoBehaviour { public float radius = 5.0F; public float power = 10.0F; public float lift = 30; public float speed = 10; public bool explode = false; public GameObject explosionParticle; void FixedUpdate() { if(Input.GetKey(KeyCode.UpArrow)){ transform.Translate(Vector3.forward * speed * Time.deltaTime); } if(explode){ Vector3 explosionPos = transform.position; Collider[] colliders = Physics.OverlapSphere(explosionPos, radius); foreach (Collider hit in colliders) { if (hit.rigidbody) { hit.rigidbody.AddExplosionForce(power, explosionPos, radius, lift); Instantiate(explosionParticle, transform.position, transform.rotation); } } } } void OnCollisionEnter(Collision collision) { if(collision.gameObject.tag == "Cube"){ explode = true; } } void OnCollisionExit(Collision collision) { if (collision.gameObject.tag == "Cube") { Destroy(gameObject); } } } |
For the new, we add explosionEffect GameObject, Instantiate after hit “Cube” tag objects and we should destroy the object so we add OnCollisionExit() method for destroying. Go to look the Bomb Inspector panel and you’ll see an empty Explosion Effect parameter and we drag and drop explosion object that we made prefab before. So we can see.
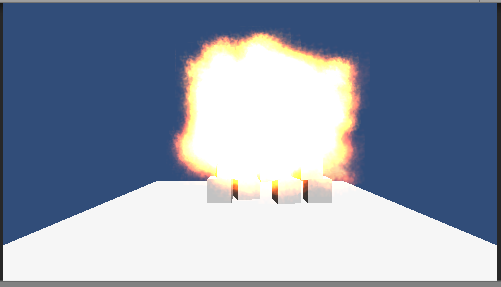
Explode !
If you don’t see the lift, you can delete the following script in the ExplodeExample below :
void OnCollisionExit(Collision collision)
{
if (collision.gameObject.tag == “Cube”)
{
Destroy(gameObject);
}
}
{
if (collision.gameObject.tag == “Cube”)
{
Destroy(gameObject);
}
}
So that’s it. If we delete the following script, the explosion will do looping Instantiate. This tutorial is not detail enough, even my english so If having a question you can comment. And for the first time, we can also play this song. See you next post 
